Your ability to read C code and identify with the perils it exposes developers to is a critical component of this course. This chapter serves as an optional 'review' to strengthen your understanding of C fundamentals, with a particular focus on the language features we find most important for this course.
The lessons offered by this chapter help smoothen the learning curve of reverse engineering, and the rest of the course.- A review of exploitation-relevant C language concepts
- Program Input and Output
- Mapping C variables to low-level memory layout
- Integer Types, Arithmetic, and Bitwise operations
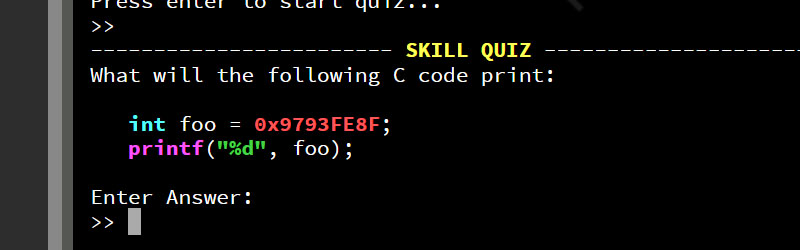